What is Flutter?
Flutter is a UI kit from Google that supports cross-platform development for Android, iOS, Web, and desktop applications. Flutter was first released to the public in 2017.
The language of the framework is Dart, which builds on today’s modern foundations. It is very similar to C# and Java, so it is easy to learn.
Everything is a Widget!
In Flutter (almost) everything is a widget. A widget is a UI component (a view) that assembles the interface as building blocks. In the Flutter framework, we have to describe the UI declaratively, in a so-called similar to widget tree. Of course, in reality our situation is much simpler than that 🙂
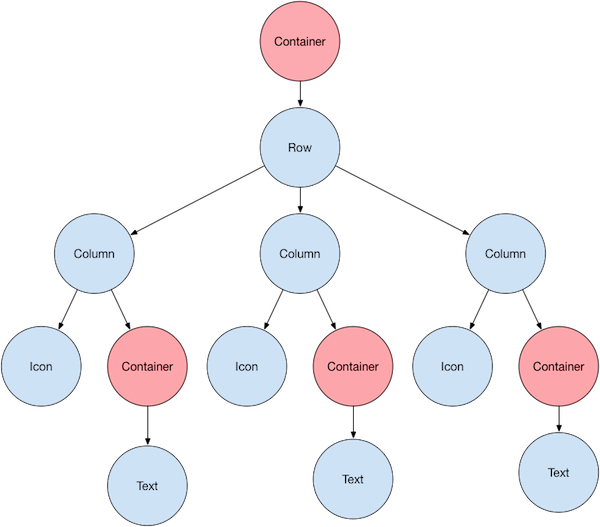
Every single pixel of the screen can be modified according to our own needs. It does not build on native OS UI components, instead we get a completely free hand in their design.
Stateful vs Stateless widget
There are two basic types of widgets: Stateless and Stateful widgets.
Stateless widget : immutable object. It’s similar to a drawing board: once it’s there, it doesn’t change.
Stateful widget : the state and thus the appearance of a Stateless object can be changed through methods. The built-in method is a call to setState().
About Flutter State management. But that’s not the point!
The appearance of a Flutter mobile application can be described by a state:
UI = f(states)
In the Flutter developer circles, there is a lot of debate about which is the “best” “State management” solution, and it causes heated debates, but this is not the most important thing!
It is very important to understand state management, however, for a small application, the existing options provided by the framework (StatefulWidget) are sufficient.
Let’s code!
The goal is to see the strengths and possibilities of Flutter through a simple app.
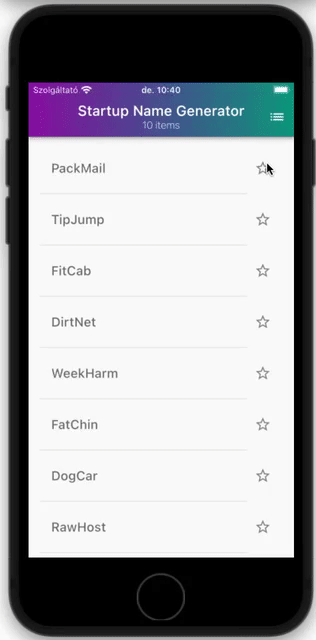
GitHub repo: https://github.com/vborbely/english_words
The Flutter Codelab I used for the demo:
- Simple ListView with details screen:
Structure of the code:
- lib directory: all code written in Dart will be organized under: business logic, UI, Helpers, etc.
- pubspec.yaml file: version-tracked printout of the packages used by the mobile app; generators; location of other dependencies
- android, ios, web, macos, linux, windows libraries: everything you need for a native build; native code snippets (we will rarely need it)
- main.dart file : default entry point of the app
Let’s test it!
Unit testing
It is the most basic part of testing. Mockito package can be used for it.
Integration testing
Its purpose is to test the entire application or a large part of it. The purpose of testing is to make sure that widgets and services (BackEnd, APIs, etc.) work well together.
The test is usually run on a real device or in an emulator (iOS Simulator, Android Emulator) to eliminate framework biases.
Integrates with Firebase Test Lab for automation .
Widget (Component) testing
We can test a widget with it.
There are 2 ways to test the widget:
- simple widget test, when we can test the existence of a UI element.
For example, is there a text widget in which the value of a counter is 2 - Based on Golden sample image file. This gives us the opportunity to pixel-accurately test our app. It compares the images created from the UI interface created during the test to a pre-generated sample image.
It marks the faulty details by visualizing them and thus making their evaluation easier.
Testing sample app: https://github.com/vborbely/bdd_showcase_app
We learned these
- Pulling in and importing package dependencies
- File organization is especially important as the app grows
- If possible, follow the 1 Widget – 1 file pattern. If possible, use a widget more than once
- use the
const
andfinal
keywords wherever possible - let’s pay attention to the specific parts of the platform. For example, there is no physical back button on iOS , so the navigation stack must be managed (back opens)
- automated testing can be done in a variety of ways
Tooling
IDEs
All major IDEs have a number of additional plugins for Flutter and Dart installed.
- JetBrains IntelliJ IDEA / Android Studio
- VSCode
CodePen
CI / CD processes
- Bitrise.io (HUN)
- CodeMagic.io from NeverCode
- GitHub Actions
Frequently used commands
Some basic commands you may need on a daily basis. The good news is that you won’t need more than that.
Command | Operation |
---|---|
flutter doctor | lists the configurations of the development environment |
flutter clean | deletes the cache files associated with the build |
flutter pub get | reloads dependencies, builds cache files |
flutter pub run build_runner build --delete-conflicting-outputs | runs the generators that create the classes. It completely regenerates the classes |
flutter pub run build_runner watch | etc., as above, but only searches for changes and updates them |
flutter build [appbundle | ios ] | creates a binary file from the project for Android or iOS |
flutter run -d | starts the app in release mode on the device, depending on the setting after the -d switch |
flutter pub add | adding a package dependency to the pubspec.yaml file |
Common Flutter CLI Commands
Useful links
- https://flutter.dev The official website of Flutter
- https://docs.flutter.dev/get-started/install Installation guide
- https://pub.dev Flutter/Dart collection site for open source packages
- https://codepen.io/pen/editor/flutter
- https://dartpad.dev
My other articles on Flutter
- A company manager who wishes he had switched to cross platform earlier (HU)
- iOS mobile developer with Flutter framework (HU)
- Creating a Chrome browser extension using Flutter UI kit (HU)
- By 2022, the native mobile application development method will be at a competitive disadvantage (HU)
- 4 compelling reasons why Flutter development is worth it now (HU)